A common question is "Should I use Next.js or React?", which is interesting considering that Next.js is built on top of React.
To clear up this confusion, let's look at the key differences between using a server-side rendered framework like Next.js and a single-page application (SPA) system like React with Vite or Create React App.
Comparing Next.js and Vite Applications
To illustrate the differences, let's take a look at two versions of the same application: one built with Next.js and the other with Vite.
The Next.js application is on port 3000, and the Vite application is on port 5173.
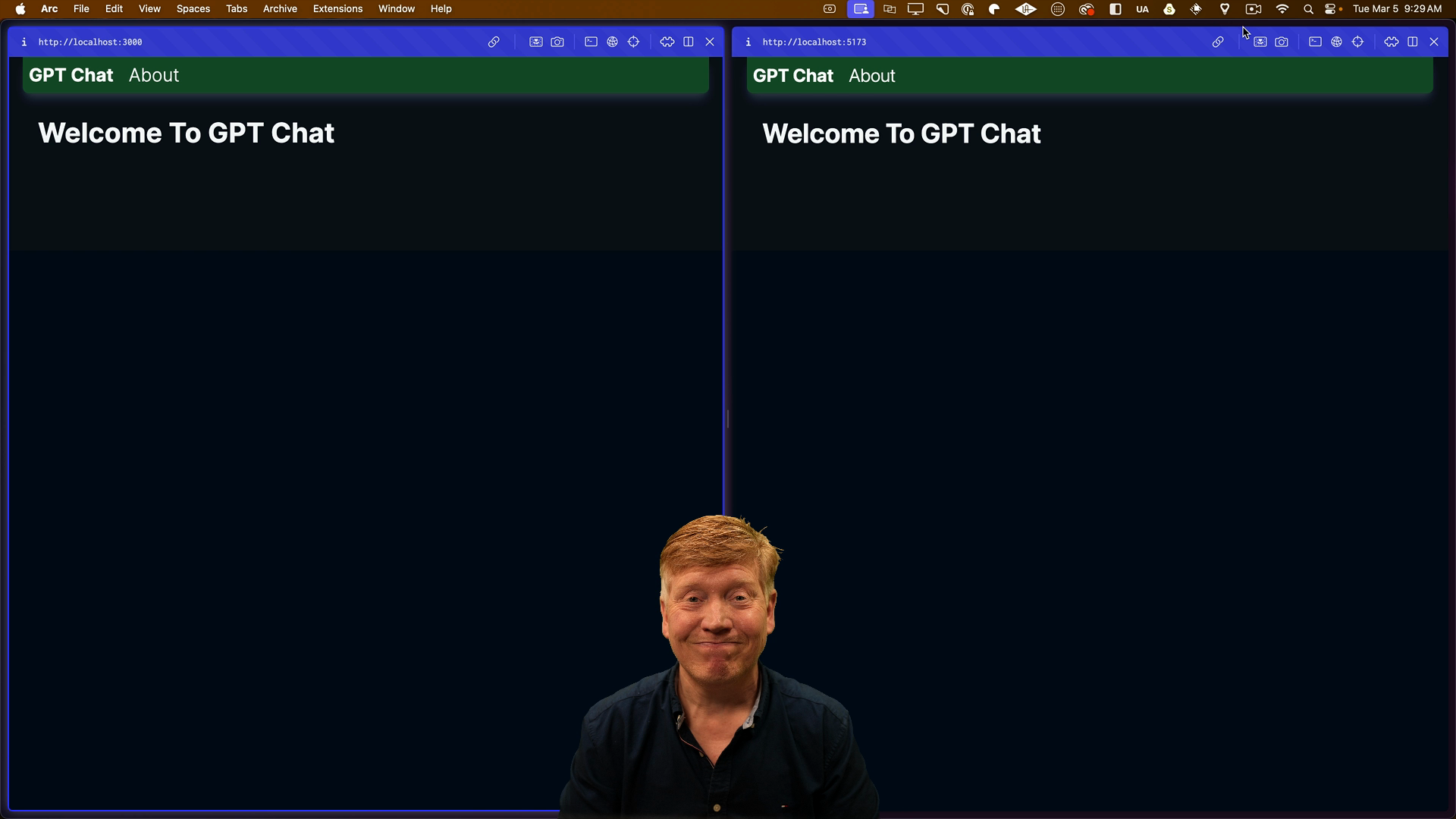
At first glance, they may look identical, but there's a significant difference under the hood.
Disabling JavaScript
To showcase the differences, we'll disable JavaScript in both applications using the developer tools. Here's how you can do it:
- Open the developer tools in your browser.
- Press Command + Shift + P (or Ctrl + Shift + P on Windows).
- Type "JavaScript" and select the option to disable JavaScript.
After disabling JavaScript and refreshing both applications, you'll notice a striking difference:
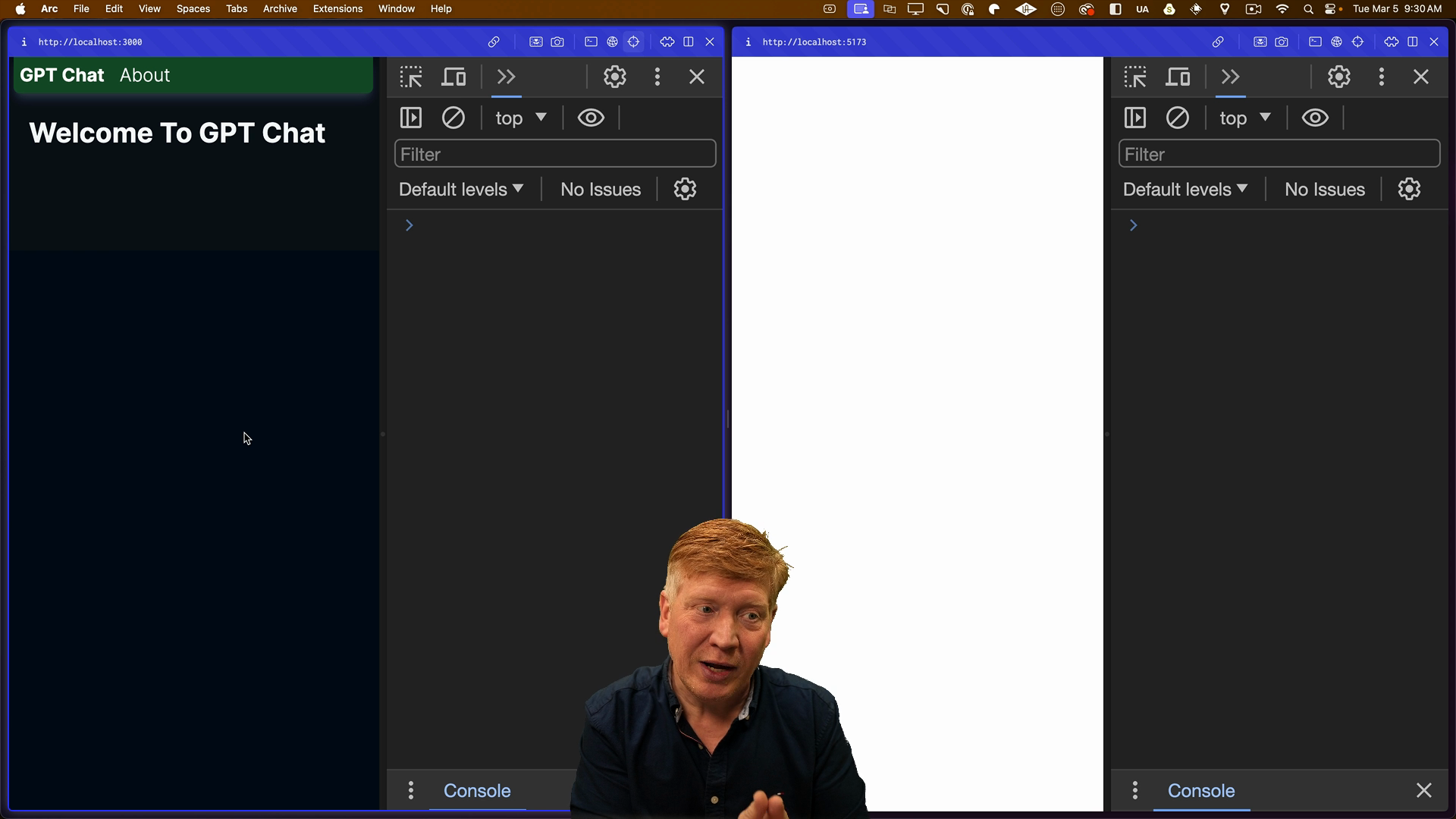
The Next.js application still shows the content, as it's server-side rendered and the HTML is already present in the page.
In contrast, the Vite application displays a blank page because it relies on JavaScript to run the React application.
Examining the Page Source
Let's take a closer look at the page source of both applications:
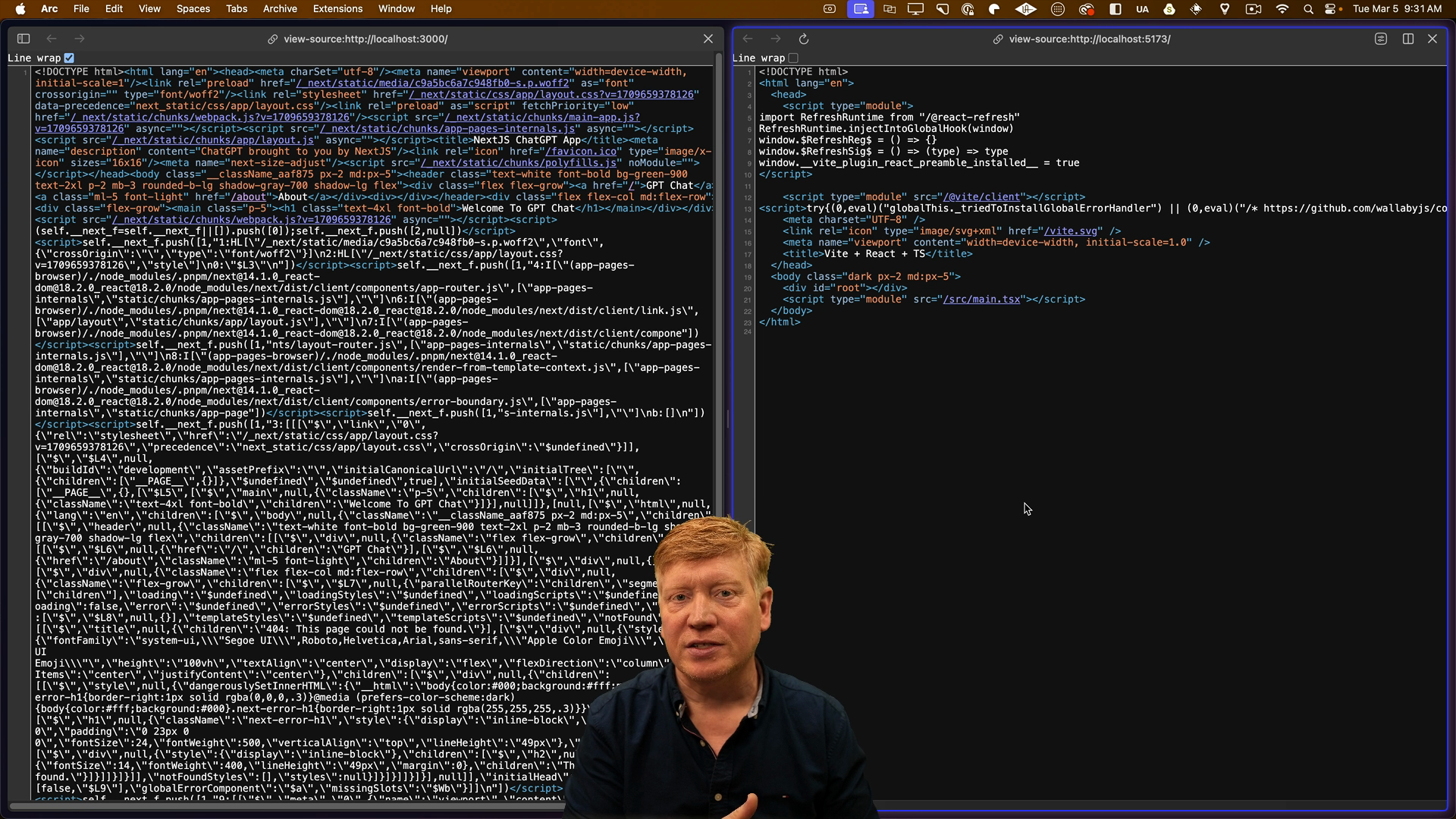
The Next.js application has a significantly larger page source compared to the Vite application, containing HTML from the start.
When you make a request to the Next.js server, it performs the following steps:
- Runs the application on the server.
- Generates the HTML.
- Sends the HTML to the client along with a script tag containing the React code bundle.
The React code is then executed on the client and hydrated, syncing the server-rendered HTML with the client-side application. This approach ensures that the user sees content on the screen quickly, which is beneficial for both the user experience and search engine optimization (SEO).
On the other hand, the Vite application has a simple structure with a div containing an ID of "root" and a script tag bringing in the application source:
<div id="root"></div>
<script type="module" src="/src/main.tsx"></script>
When JS is enabled, the client brings in the source for the application, mounts it onto the root component, and renders the application on the client-side.
SEO Considerations
Search engines like Google analyze the HTML output of servers to determine the content of a webpage. While there are varying opinions on whether search engines execute client-side JavaScript, many SEO experts recommend server-side rendering to ensure that the desired HTML is directly sent to search engine bots.
By server-side rendering your application, you provide search engines with the complete HTML content, rather than relying on them to execute JavaScript to render the page.
Understanding Client Components in Next.js
In Next.js, you can create client components that allow for interactivity on the client-side. Let's create a simple example to demonstrate how client components work:
"use client"
export default function ClientComponent() {
return <div>HELLO FROM THE CLIENT</div>
}
The 'use client'
directive at the top of the file ensures that the component runs on both the client and the server.
We can then import the ClientComponent
in the page.tsx
file:
import ClientComponent from "./ClientComponent"
export default function Home() {
return (
<main className="p-5">
<h1 className="text-4xl font-bold">Welcome to GPT Chat</h1>
<ClientComponent />
</main>
)
}
After importing and using the ClientComponent
in your application, you'll see the message rendered on the page:
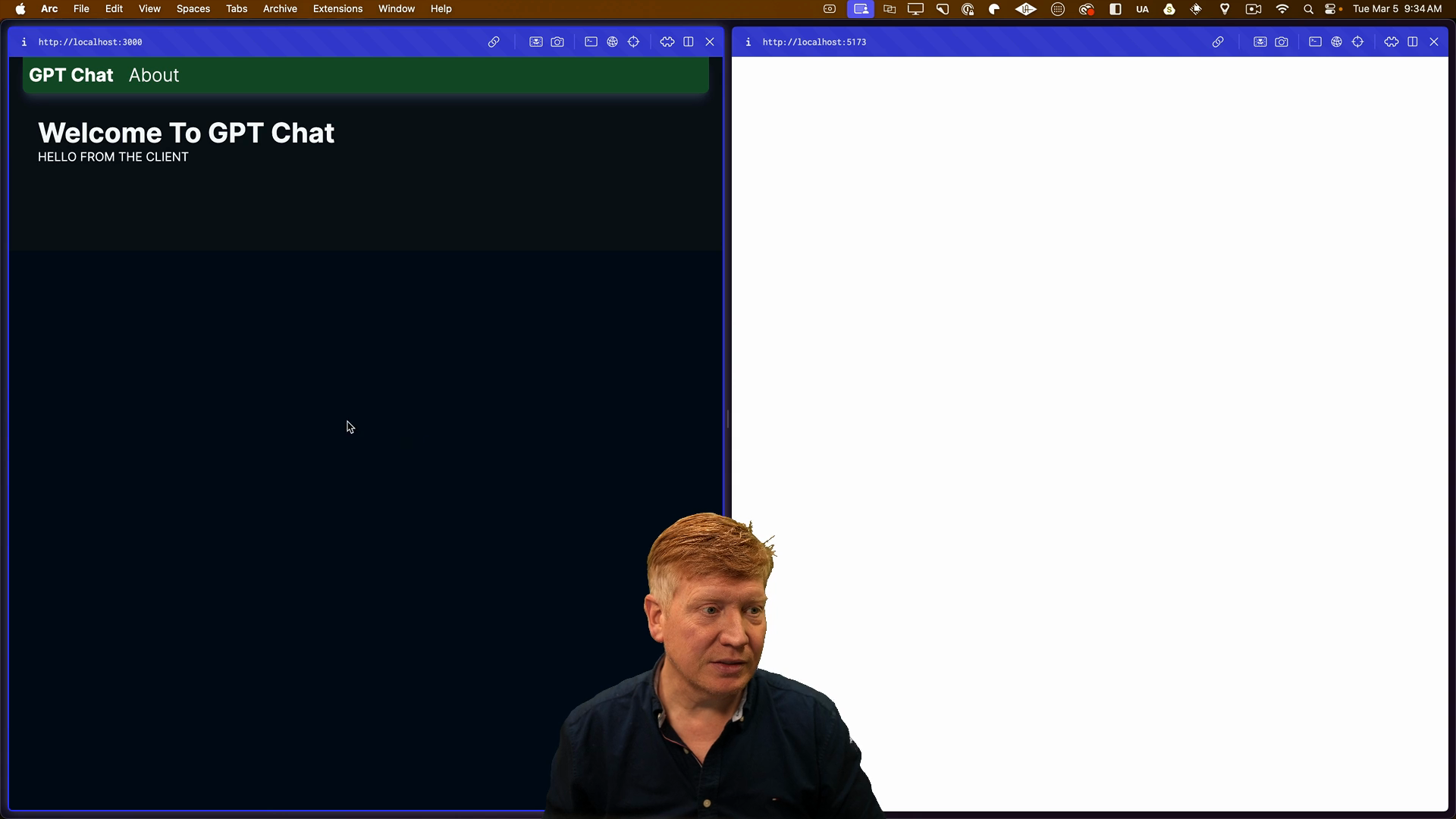
Looking at the source of the page, the rendered HTML from the server includes the content of the client component:
This demonstrates that client components in Next.js run on both the client and the server, contrary to the common misconception that they only run on the client-side.
With this misconception cleared up, let's continue on with adding authorization to the app.