Configure Container Queries with Tailwind CSS

The first step for creating the layout is to add the following classes to the main container:
<main className="mx-auto max-w-[960px] flex flex-wrap">
<!-- ... -->
</main>
These classes will:
- Center the container horizontally with
mx-auto
- Set a maximum width with
max-w-[960px]
- Use a flex layout with
flex
- Allow the flex items to wrap with
flex-wrap
Here's how the page should look now:
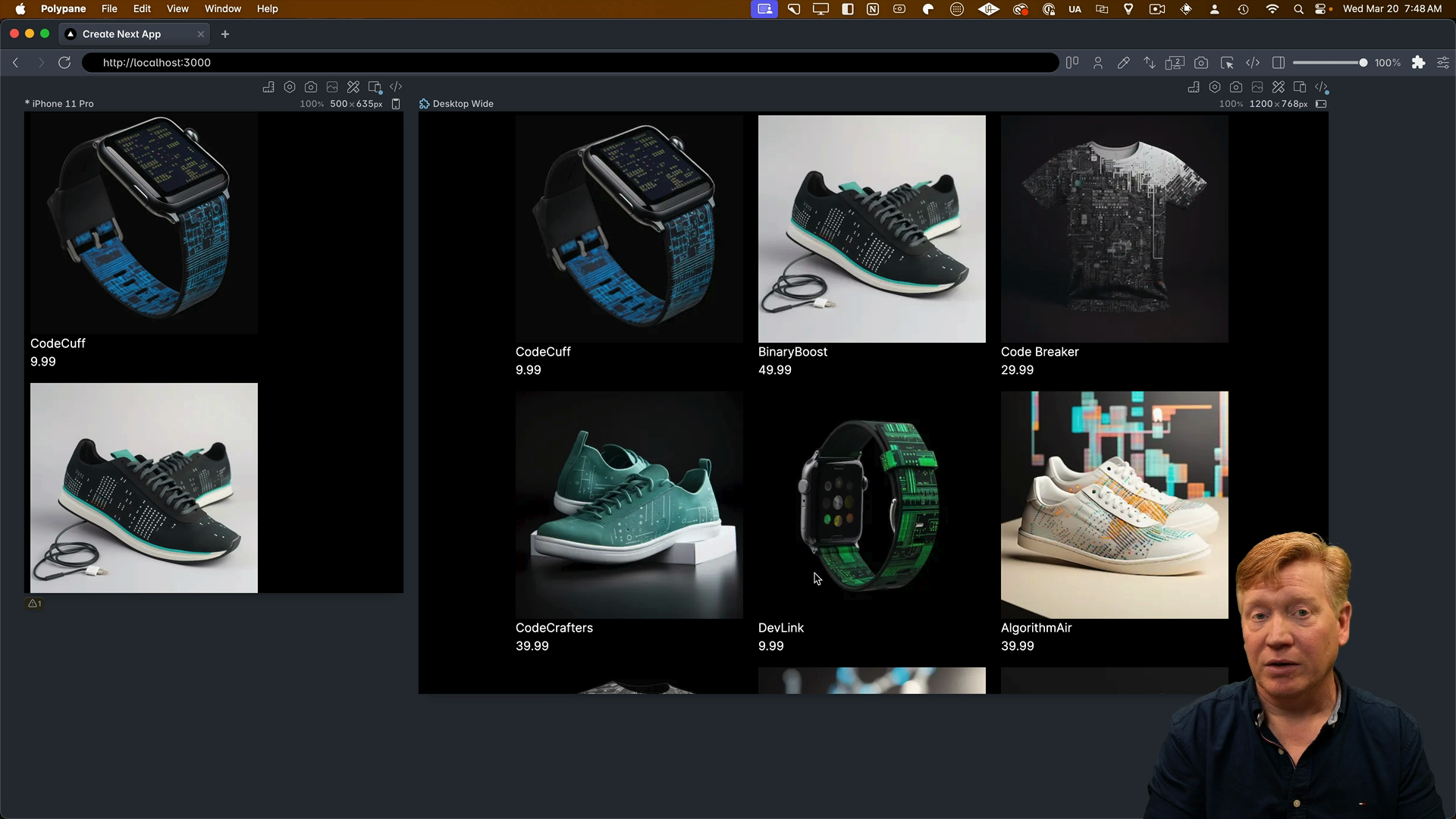
If you're curious about why we're using these specific classes, revisit the CSS modules lessons where we dive into each of these CSS choices in detail.
Adding Product Card Styles
Inside of the ProductCard.tsx
component file, we'll add the @container
class to the wrapping div to enable container queries:
export const ProductCard = ({ product }: Props) => {
return (
<div className="@container">
...
In order to see the container query in action, we need to change the interior div from a flex
row-based layout to a column-based layout. We can do this by adding the following classes:
// Inside the ProductCard component
<div className="@container">
<div className="flex flex-col @md:flex-row">
<div>
<Image
...
>
This will use a column-based layout by default but switch to a row-based layout on medium-sized screens and above.
However, since we're using Tailwind version 3, we'll need to add a plugin for container queries.
Browser Support for Container Queries
To add the container queries plugin, we need to stop the development server, then install the plugin:
pnpm add -D @tailwindcss/container-queries
Then inside of the tailwind.config.js
file, we'll add the plugin:
module.exports = {
content: ["./src/**/*.{js,ts,jsx,tsx,mdx}"],
theme: {
extend: {},
},
plugins: [require("@tailwindcss/container-queries")],
};
After restarting the dev server, we can see the horizontal layout on small screens and the vertical layout on medium screens and above:
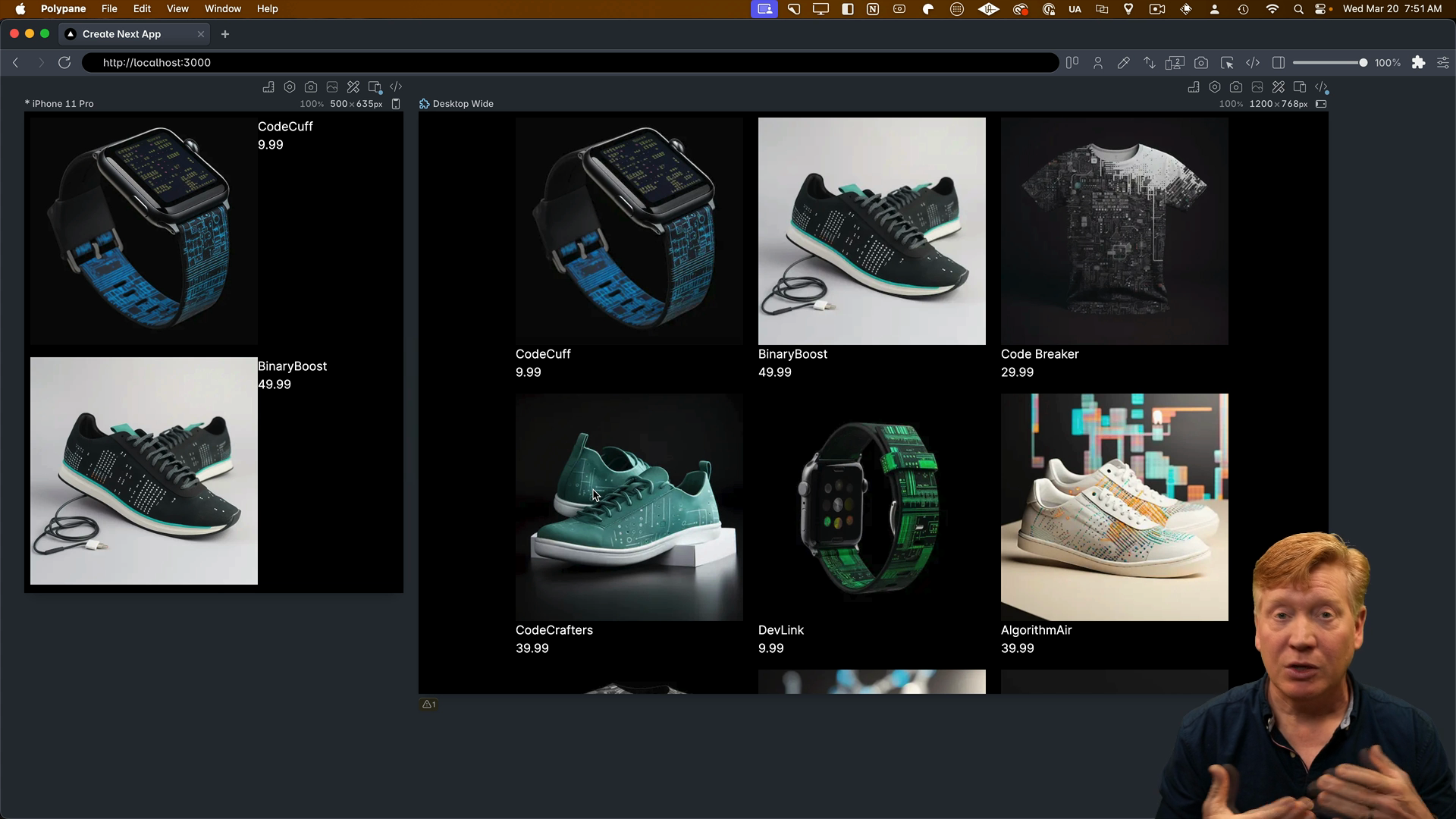
In Tailwind version 4, container queries are built-in, so you won't need to do this extra step. Thank you, Tailwind v4, for making our lives easier!
Also, if you're worried about browser support for container queries, don't be. Globally, 91.33% of browsers support them according to Can I Use. This means you can use container queries in most projects without any issues.
Finishing the Product Card
To finish up the product card, we'll add a few more classes to the image and info container.
The w-full h-auto
classes will make the image take up the full width of the card in the vertical layout but only 25% of the width in the horizontal layout. The rounded-
classes will add some rounding to the corners of the image:
export const ProductCard = ({ product }: Props) => {
return (
<div className="@container">
<div className="flex flex-col @md:flex-row">
<div className="w-full @md:w-1/4">
<Image
src={product.image}
alt={product.title}
width={300}
height={300}
className="w-full h-auto rounded-tl-2xl rounded-tr-2xl @md:rounded-tr-none rounded-bl-none @md:rounded-bl-2xl"
/>
</div>
...
With these final changes, our product card is complete! It looks fantastic and handles both light and dark mode.
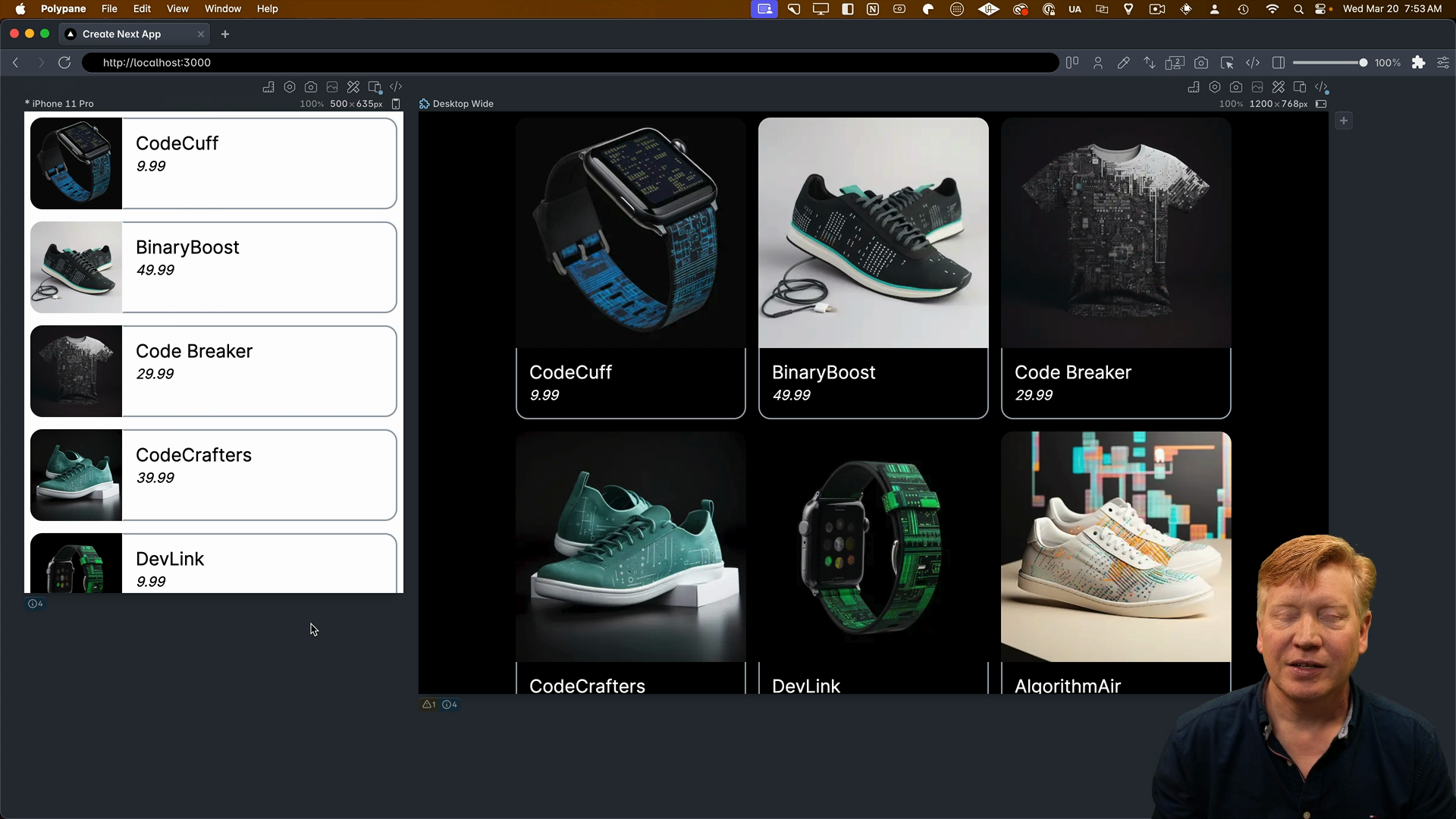
Using Tailwind with CSS Modules
In the next video, we'll explore a variation of how to use Tailwind that combines CSS modules with Tailwind using the @apply
directive.