Playwright is another option for running E2E tests in your Next.js application. Let's take a look at how it works.
Initial Project Setup
Like before, we'll create a new Next.js Application with the following command:
pnpm dlx create-next-app@latest e2e-with-playwright --use-pnpm
Next, install Playwright and configure it for our project using the following command:
pnpm create playwright
This command will guide you through a setup process:
binaries for Chromium, Firefox, and WebKit, enabling us to test our application across different browsers.
Choose to install Playwright in the e2e
directory within your src
folder. You can skip the GitHub Actions workflow setup for now. However, make sure to select the option to "install the Playwright browsers" as this is essential for running our E2E tests!
Configuring Playwright for Next.js
With Playwright installed, we need to make a few adjustments to the configuration.
Open the playwright.config.ts
file. First, we'll enable the automatic launch of our development server when running E2E tests. Uncomment the following line and set the command
to npm run dev
:
// inside playwright.config.ts
/* Run your local dev server before starting the tests */
webServer: {
command: "npm run dev",
url: "http://127.0.0.1:3000",
reuseExistingServer: !process.env.CI,
},
Next, we need to specify the base URL for our tests. This ensures that relative paths used in our tests resolve to the correct location. Update the baseURL
property to http://127.0.0.1:3000
as shown below:
// inside playwright.config.ts
/* Shared settings for all the projects below. See https://playwright.dev/docs/api/class-testoptions. */
use: {
/* Base URL to use in actions like `await page.goto('/')`. */
baseURL: "http://127.0.0.1:3000",
/* Collect trace when retrying the failed test. See https://playwright.dev/docs/trace-viewer */
trace: "on-first-retry",
},
Create a Basic E2E Test
Before writing our first test, let's modify our Next.js application to have some content we can test against. In the app/page.tsx
file, replace the existing code with the following:
// inside app/page.tsx
export default async function Home() {
const pokemon = (await fetch("https://pokeapi.co/api/v2/pokemon").then(
(res) => res.json()
)) as { results: { name: string }[] };
return (
<div className="bg-black text-white p-5">
<h1>Pokemon</h1>
<ul>
{pokemon.results.map((p) => (
<li key={p.name}>{p.name}</li>
))}
</ul>
</div>
);
}
As before, this component will render a basic list of Pokemon names fetched from the PokeAPI.
Now, navigate to the e2e
directory and delete the example.spec.ts
file. Create a new file named home.spec.ts
and add the following code:
import { test, expect } from "@playwright/test";
test("simple home page test", async ({ page }) => {
await page.goto("/");
await expect(page.getByText("bulbasaur")).toBeVisible();
});
This test navigates to the root of our application (/
) and checks if the text "bulbasaur" is visible on the page.
Running E2E Tests
We can now execute our test using the following command:
pnpm exec playwright test
Playwright will launch the headless versions of Chromium, Firefox, and WebKit, run the test in each browser, and display the results in the terminal.
After the tests finish, you can get a more detailed report with the following command:
pnpm exec playwright test
This will open a report in your browser, providing information about each test run, including screenshots and step-by-step actions.
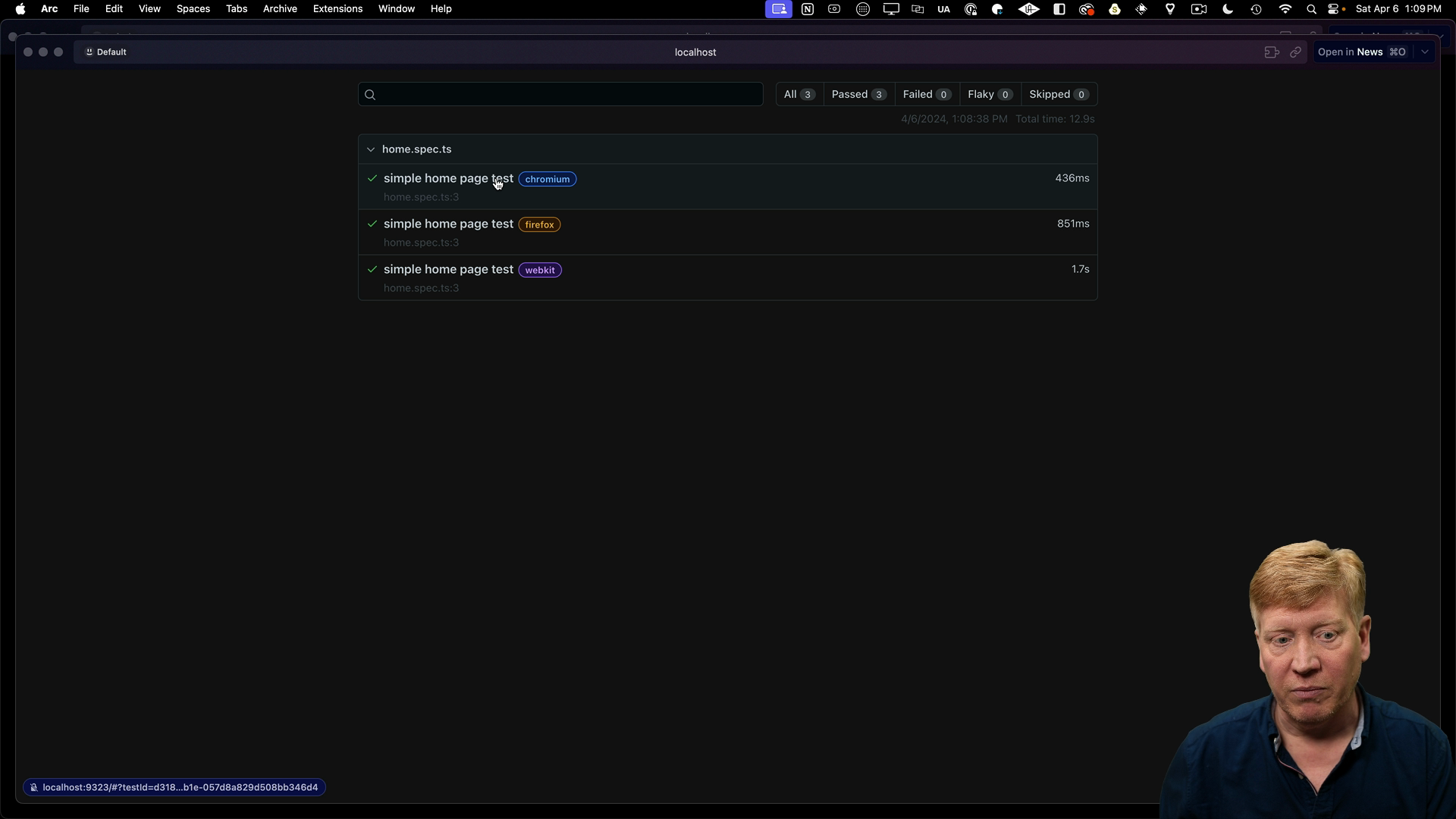
Finally, to simplify running our E2E tests, we can add a script to our package.json
file:
// inside package.json
"scripts": {
// ... other scripts
"test:e2e": "playwright test"
},
Now, we can run our E2E tests using npm run test:e2e
.